Forgot bit locker pin, forgot bit locker recovery key, 5 Easy ways to fix
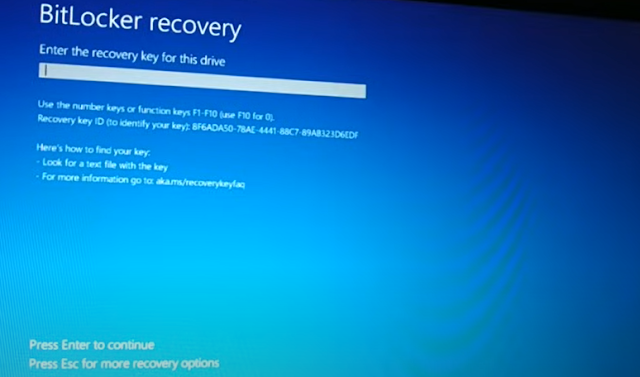
Python syntax refers to the set of rules and structure that defines how a Python program is written and interpreted. Understanding Python syntax is essential to writing code that the Python interpreter can execute. Below is an overview of Python syntax and how to use it:
### 1.
**Basic Syntax**
- **Case Sensitivity**: Python is
case-sensitive, so `Variable` and `variable` are considered different.
- **Indentation**: Indentation is used to
define blocks of code. Python uses indentation (usually four spaces or a tab)
to indicate a block of code, such as in loops, conditionals, and function
definitions. For example:
```python
if condition:
# Indented block
print("Condition is true")
```
- **Comments**: Comments are lines that
Python ignores, used for annotations. Single-line comments start with `#`:
```python
# This is a comment
print("Hello, World!") # This prints Hello, World!
```
- **Multi-line Comments**: Use triple quotes
(`'''` or `"""`) for multi-line comments:
```python
"""
This is a
multi-line comment.
"""
```
### 2.
**Variables and Data Types**
- **Variable Assignment**: Variables do not
need explicit declaration to reserve memory space. The assignment happens
automatically when a value is assigned to a variable.
```python
x = 5
name = "Alice"
```
- **Data Types**: Python has several
built-in data types such as:
- `int` (integer): `x = 5`
- `float` (floating-point number): `y =
3.14`
- `str` (string): `name =
"Alice"`
- `bool` (boolean): `is_true = True`
- `list`: `numbers = [1, 2, 3]`
- `dict`: `student = {"name":
"Alice", "age": 25}`
### 3.
**Operators**
- **Arithmetic Operators**: `+`, `-`, `*`,
`/`, `%`, `**` (exponentiation), `//` (floor division).
```python
result = 10 + 5 # 15
```
- **Comparison Operators**: `==`, `!=`,
`>`, `<`, `>=`, `<=`.
```python
is_equal = (10 == 10) # True
```
- **Logical Operators**: `and`, `or`, `not`.
```python
result = (10 > 5) and (5 < 10) # True
```
- **Assignment Operators**: `=`, `+=`, `-=`,
`*=`, `/=`, etc.
```python
x = 5
x += 3
# x is now 8
```
### 4.
**Control Flow**
- **If Statements**: Used to make decisions.
```python
if condition:
# code block
elif another_condition:
# another block
else:
# else block
```
- **For Loops**: Used to iterate over a
sequence (like a list, tuple, dictionary, set, or string).
```python
for i in range(5):
print(i)
```
- **While Loops**: Used to execute a set of
statements as long as a condition is true.
```python
while condition:
# code block
```
### 5.
**Functions**
- **Defining a Function**: Use the `def`
keyword.
```python
def my_function():
print("Hello from a
function")
```
- **Calling a Function**:
```python
my_function()
```
- **Function Parameters**:
```python
def greet(name):
print(f"Hello, {name}")
greet("Alice")
```
- **Return Statement**: Use `return` to
return a value from a function.
```python
def add(a, b):
return a + b
result = add(5, 3)
```
### 6.
**Data Structures**
- **Lists**: Ordered, mutable collection of
items.
```python
fruits = ["apple",
"banana", "cherry"]
```
- **Tuples**: Ordered, immutable collection
of items.
```python
colors = ("red",
"green", "blue")
```
- **Dictionaries**: Unordered collection of
key-value pairs.
```python
student = {"name":
"Alice", "age": 25}
```
- **Sets**: Unordered collection of unique
items.
```python
unique_numbers = {1, 2, 3, 4}
```
### 7.
**Modules and Libraries**
- **Importing Modules**: Use `import` to include
modules.
```python
import math
print(math.sqrt(16))
```
- **From Import**: Import specific functions
or variables from a module.
```python
from math import sqrt
print(sqrt(16))
```
### 8.
**File Handling**
- **Opening and Closing Files**:
```python
file = open("example.txt",
"r")
content = file.read()
file.close()
```
- **With Statement**: Automatically closes
the file.
```python
with open("example.txt",
"r") as file:
content = file.read()
```
### 9.
**Error Handling**
- **Try-Except Block**: Used to handle
exceptions.
```python
try:
result = 10 / 0
except ZeroDivisionError:
print("Cannot divide by
zero")
```
### 10.
**Classes and Objects**
- **Defining a Class**:
```python
class Dog:
def __init__(self, name, breed):
self.name = name
self.breed = breed
def bark(self):
print(f"{self.name} says
woof!")
my_dog = Dog("Buddy",
"Golden Retriever")
my_dog.bark()
```
###
Conclusion
Python
syntax is designed to be readable and intuitive, making it easier for beginners
to start coding. By understanding and applying the basic syntax rules, you can
write effective Python code for various applications.