Forgot bit locker pin, forgot bit locker recovery key, 5 Easy ways to fix
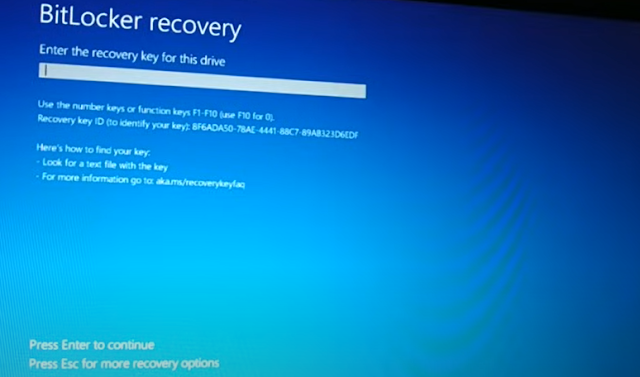
### Python User Input: An Overview
In Python,
**user input** refers to data provided by the user during the execution of a
program. Python provides a built-in function called **`input()`** to capture
user input from the console. This input is then stored as a string, which can
be processed or converted to other data types.
### Basic
Usage of `input()`
#### Syntax:
```python
variable =
input(prompt)
```
-
**`prompt`**: A string that is displayed to the user as a prompt (optional).
-
**`variable`**: Stores the input entered by the user.
### Example:
Basic User Input
```python
name =
input("Enter your name: ")
print(f"Hello,
{name}!")
```
**Output:**
```
Enter your
name: John
Hello, John!
```
Here, the
**`input()`** function displays the prompt **"Enter your name: "**
and waits for the user to type something. Once the user presses **Enter**, the
input is stored in the variable `name`, which is then used in the `print()` statement.
### Handling
User Input as Different Data Types
By default,
`input()` captures input as a **string**. If you need to work with numeric
values or other data types, you’ll need to convert the string to the desired
type using functions like **`int()`**, **`float()`**, or **`bool()`**.
#### Example
1: Converting Input to an Integer
```python
age =
int(input("Enter your age: "))
print(f"Next
year, you will be {age + 1} years old.")
```
**Output:**
```
Enter your
age: 25
Next year,
you will be 26 years old.
```
Here, the
input is converted to an integer using the `int()` function, allowing you to
perform arithmetic operations.
#### Example
2: Converting Input to a Float
```python
weight =
float(input("Enter your weight in kilograms: "))
print(f"Your
weight is {weight} kg.")
```
**Output:**
```
Enter your
weight in kilograms: 70.5
Your weight
is 70.5 kg.
```
#### Example
3: Handling Boolean Input
```python
is_student =
input("Are you a student? (yes/no): ").lower() == "yes"
print(f"Student
status: {is_student}")
```
**Output:**
```
Are you a
student? (yes/no): yes
Student
status: True
```
In this
case, the user input is compared to the string "yes", and a boolean value
is stored in `is_student`.
### Validating
and Handling Invalid Input
Since user
input can be unpredictable, you should validate it to avoid errors (e.g.,
entering non-numeric data when expecting numbers).
####
Example: Handling Invalid Input with `try-except`
```python
try:
num = int(input("Enter a number:
"))
print(f"You entered the number
{num}.")
except
ValueError:
print("Error: Please enter a valid
number.")
```
**Output
(Invalid Input):**
```
Enter a
number: hello
Error:
Please enter a valid number.
```
In this
example, if the user enters something that can’t be converted to an integer, a
**`ValueError`** is raised, and the exception is caught by the `except` block.
### Example:
Handling Multiple Inputs
You can ask
for multiple inputs in one line by using the `split()` method:
```python
name, age =
input("Enter your name and age, separated by a space: ").split()
print(f"Name:
{name}, Age: {age}")
```
**Output:**
```
Enter your
name and age, separated by a space: John 25
Name: John,
Age: 25
```
Here,
`split()` divides the input string into a list of values based on spaces, and
these values are assigned to the variables `name` and `age`.
### Example:
Using a Default Input Value
You can
predefine a default value for an input by using a conditional expression:
```python
name =
input("Enter your name (or press Enter to use 'Guest'): ") or
"Guest"
print(f"Welcome,
{name}!")
```
**Output:**
```
Enter your
name (or press Enter to use 'Guest'):
Welcome,
Guest!
```
If the user
presses **Enter** without providing input, the variable `name` gets the value
**"Guest"**.
### Best
Practices for Working with User Input
1. **Input
Validation**: Always validate user input, especially when expecting specific
types (like numbers). Use `try-except` blocks to catch errors.
2. **Data
Conversion**: Remember to convert input to the appropriate data type (e.g.,
`int()`, `float()`, etc.) when needed.
3. **Provide
Clear Prompts**: Make sure your prompts are clear and instruct the user on what
kind of input you expect.
4. **Handle
Empty Input**: Plan for scenarios where the user provides no input, and handle
them gracefully using default values or validation.
### Example:
Full Program with Input Validation
```python
def
get_user_age():
while True:
try:
age = int(input("Please enter
your age: "))
if age < 0:
print("Age cannot be
negative. Try again.")
else:
return age
except ValueError:
print("Invalid input. Please
enter a number.")
name =
input("Enter your name: ")
age =
get_user_age()
print(f"Hello,
{name}. You are {age} years old.")
```
In this
example, a helper function **`get_user_age()`** keeps prompting the user until
valid input (a non-negative integer) is provided.