Forgot bit locker pin, forgot bit locker recovery key, 5 Easy ways to fix
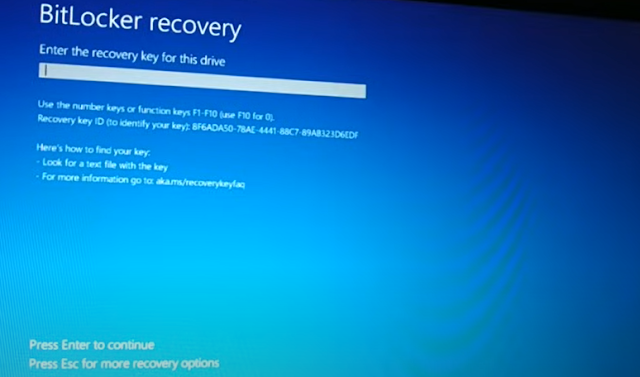
Creating a web-based GUI (Graphical User Interface) in Python can be accomplished using web frameworks like Flask or Django. These frameworks allow you to build web applications that serve HTML, CSS, and JavaScript to create interactive and user-friendly interfaces.
Here’s a
step-by-step guide to creating a simple web GUI in Python using Flask:
### 1.
**Install Flask**
First, you
need to install Flask. This can be done using pip:
```bash
pip install
Flask
```
### 2.
**Create the Project Structure**
Create a
directory for your project and navigate into it:
```bash
mkdir
my_web_gui
cd
my_web_gui
```
Inside this
directory, create the following structure:
```
my_web_gui/
│
├── app.py
├── templates/
│ ├── index.html
│ └── result.html
└── static/
└── style.css
```
-
**`app.py`**: The main Python file where your Flask application logic will
reside.
-
**`templates/`**: Directory to store HTML templates.
-
**`static/`**: Directory to store static files like CSS, JavaScript, and
images.
### 3.
**Write the Flask Application**
In `app.py`,
write the following code to create a simple Flask application with a web GUI:
```python
from flask
import Flask, render_template, request
app =
Flask(__name__)
@app.route("/")
def index():
return
render_template("index.html")
@app.route("/result",
methods=["POST"])
def
result():
# Retrieve data from the form
user_input =
request.form.get("user_input")
processed_data = f"Hello,
{user_input}!"
return
render_template("result.html", result=processed_data)
if __name__
== "__main__":
app.run(debug=True)
```
-
**`@app.route("/")`**: The main route that serves the index page with
a form.
-
**`@app.route("/result", methods=["POST"])`**: A route that
processes the form data and displays the result.
### 4.
**Create HTML Templates**
####
**index.html**
Create a
basic HTML form in `templates/index.html`:
```html
<!DOCTYPE
html>
<html
lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Web GUI Example</title>
<link rel="stylesheet"
href="{{ url_for('static', filename='style.css') }}">
</head>
<body>
<h1>Enter Your Name</h1>
<form action="/result"
method="POST">
<input type="text"
name="user_input" required>
<input type="submit"
value="Submit">
</form>
</body>
</html>
```
This
template contains a simple form where users can enter their name. The form
sends data to the `/result` route when submitted.
####
**result.html**
Create a
result page in `templates/result.html`:
```html
<!DOCTYPE
html>
<html
lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,
initial-scale=1.0">
<title>Result</title>
<link rel="stylesheet"
href="{{ url_for('static', filename='style.css') }}">
</head>
<body>
<h1>{{ result }}</h1>
<a href="/">Go
back</a>
</body>
</html>
```
This
template displays the processed data sent from the Flask route.
### 5. **Add
CSS Styling (Optional)**
Create a
simple CSS file at `static/style.css` to style your web GUI:
```css
body {
font-family: Arial, sans-serif;
text-align: center;
background-color: #f9f9f9;
margin-top: 50px;
}
h1 {
color: #333;
}
form {
margin: 20px auto;
}
input[type="text"]
{
padding: 10px;
font-size: 16px;
}
input[type="submit"]
{
padding: 10px 20px;
font-size: 16px;
background-color: #4CAF50;
color: white;
border: none;
cursor: pointer;
}
input[type="submit"]:hover
{
background-color: #45a049;
}
```
### 6. **Run
the Flask Application**
To run your
Flask application, navigate to the directory containing `app.py` and execute:
```bash
python
app.py
```
If
everything is set up correctly, Flask will start a development server, and
you’ll see output like this:
```bash
* Running on http://127.0.0.1:5000/ (Press
CTRL+C to quit)
```
Open your
web browser and go to `http://127.0.0.1:5000/` to see your web GUI.
### 7.
**Testing the Web GUI**
- Enter your
name in the text field and submit the form.
- You will
be redirected to the result page showing a personalized greeting.
### Summary
- **Flask
Installation**: Use `pip install Flask` to get started.
- **Project
Structure**: Organize your files with templates and static assets in separate
directories.
- **HTML
Templates**: Create forms and display results using HTML and Flask’s templating
engine.
- **CSS
Styling**: Style your GUI using CSS for a better user experience.
- **Run
Locally**: Start the Flask server using `python app.py` and test the
application in your browser.
This guide
walks you through creating a simple web-based GUI in Python using Flask. For
more advanced GUIs, you can integrate JavaScript frameworks like React or
Vue.js, or explore full-fledged web frameworks like Django.