Forgot bit locker pin, forgot bit locker recovery key, 5 Easy ways to fix
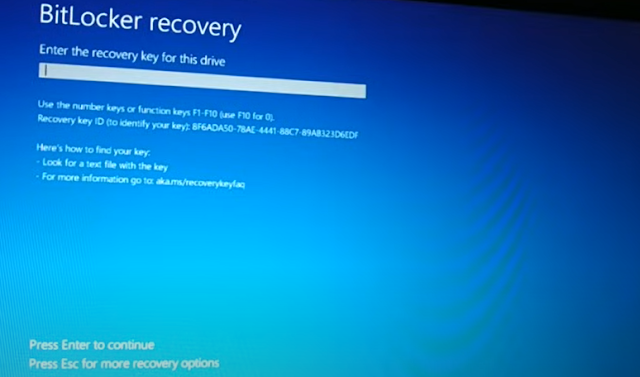
### Python Math: An Overview
In Python,
**math** typically refers to the **math module**, which provides mathematical
functions, constants, and tools for working with numbers. This module comes
pre-installed with Python, so you can easily import and use it in your code.
### Common
Uses of Python's `math` Module
1.
**Mathematical constants**:
- `math.pi`: Returns the value of pi (π ≈
3.14159).
- `math.e`: Returns the base of the natural
logarithm (e ≈ 2.71828).
2. **Basic
Mathematical Functions**:
- `math.sqrt(x)`: Returns the square root of
`x`.
- `math.pow(x, y)`: Returns `x` raised to
the power of `y` (equivalent to `x ** y`).
- `math.factorial(x)`: Returns the factorial
of `x`.
- `math.ceil(x)`: Rounds up `x` to the
nearest integer.
- `math.floor(x)`: Rounds down `x` to the
nearest integer.
- `math.gcd(x, y)`: Returns the greatest common
divisor of `x` and `y`.
3.
**Trigonometric Functions**:
- `math.sin(x)`: Returns the sine of `x`
(where `x` is in radians).
- `math.cos(x)`: Returns the cosine of `x`.
- `math.tan(x)`: Returns the tangent of `x`.
- `math.degrees(x)`: Converts radians to
degrees.
- `math.radians(x)`: Converts degrees to
radians.
4.
**Logarithmic and Exponential Functions**:
- `math.log(x, base)`: Returns the logarithm
of `x` to the given base. If no base is specified, the natural logarithm (base
`e`) is used.
- `math.exp(x)`: Returns `e` raised to the
power of `x`.
5.
**Comparison Functions**:
- `math.isclose(a, b, rel_tol, abs_tol)`:
Returns `True` if `a` is close to `b` within a tolerance.
- `math.isfinite(x)`: Checks if `x` is
finite.
- `math.isinf(x)`: Checks if `x` is
infinite.
- `math.isnan(x)`: Checks if `x` is
"Not a Number" (NaN).
### How to
Use the `math` Module
Here’s an
example demonstrating how to use the `math` module:
```python
import math
# Using
constants
print("The
value of pi is:", math.pi)
# Square
root and power
print("Square
root of 25 is:", math.sqrt(25))
print("2
raised to the power 3 is:", math.pow(2, 3))
# Factorial
print("Factorial
of 5 is:", math.factorial(5))
#
Trigonometric functions
angle_in_radians
= math.radians(30)
print("Sine
of 30 degrees is:", math.sin(angle_in_radians))
# Logarithms
print("Natural
logarithm of 10 is:", math.log(10))
print("Logarithm
of 1000 with base 10 is:", math.log(1000, 10))
```
### How to
Install the `math` Module
The `math`
module is part of Python's standard library, so no additional installation is
needed. You just need to import it at the start of your script:
```python
import math
```
If you need
more advanced mathematical functions beyond the basic `math` module, you can
explore third-party libraries like **NumPy** or **SciPy**, which provide
additional tools for numerical computations.