Forgot bit locker pin, forgot bit locker recovery key, 5 Easy ways to fix
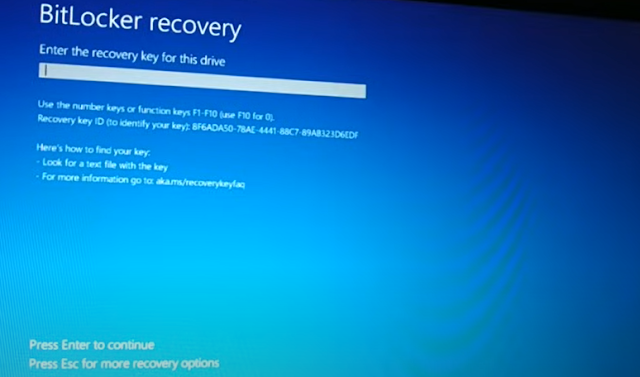
### Python `try-except`: An Overview
The
**`try-except`** block in Python is used for **exception handling**, which
allows you to handle errors gracefully without crashing the program. When a
piece of code might cause an error (an exception), you can use the `try` block
to attempt execution. If an exception occurs, Python will skip the rest of the
`try` block and run the code inside the `except` block to handle the error.
This
mechanism ensures your program can handle errors and unexpected conditions more
elegantly.
### Basic
Syntax of `try-except`
```python
try:
# Code that might raise an exception
except:
# Code to run if an exception occurs
```
### Example
of Using `try-except`
```python
try:
num = int(input("Enter a number:
"))
result = 10 / num
print(f"Result: {result}")
except
ZeroDivisionError:
print("Error: Division by zero is not
allowed.")
except
ValueError:
print("Error: Invalid input. Please
enter a valid integer.")
```
#### Output
for Invalid Input:
```
Enter a
number: hello
Error:
Invalid input. Please enter a valid integer.
```
#### Output
for Division by Zero:
```
Enter a
number: 0
Error:
Division by zero is not allowed.
```
In this
example:
- The `try`
block contains code that may raise two types of exceptions:
**`ZeroDivisionError`** (when dividing by zero) and **`ValueError`** (when
converting a non-numeric input).
- The
`except` block handles these specific exceptions.
### How to
Use `try-except` with Multiple Exceptions
You can
specify different `except` blocks for different types of exceptions:
```python
try:
# Code that might raise different
exceptions
except
TypeError:
# Handle TypeError
except
IndexError:
# Handle IndexError
except
Exception as e:
# Handle any other exception
print(f"An error occurred: {e}")
```
The
`Exception` class is the base class for all exceptions in Python, so the last
`except Exception as e` will catch any type of exception that was not caught by
the specific ones above.
### Using
`else` with `try-except`
The `else`
block runs only if no exceptions occur in the `try` block:
```python
try:
num = int(input("Enter a number:
"))
result = 10 / num
except
ZeroDivisionError:
print("Error: Division by zero.")
except
ValueError:
print("Error: Invalid input.")
else:
print(f"Result: {result}")
```
Here, the
`else` block will run only if there are no errors in the `try` block.
### Using
`finally` with `try-except`
The
`finally` block is used to define actions that should always be executed,
regardless of whether an exception occurs or not. It’s useful for cleaning up
resources (like closing a file or a database connection).
```python
try:
file = open("example.txt",
"r")
content = file.read()
print(content)
except
FileNotFoundError:
print("Error: File not found.")
finally:
file.close() # Ensure the file is closed whether an
exception occurs or not
```
In this
example, the `finally` block ensures that the file is closed no matter what
happens during the file reading.
### Catching
Multiple Exceptions in One Line
You can
catch multiple exceptions in a single `except` block by specifying them as a
tuple:
```python
try:
num = int(input("Enter a number:
"))
result = 10 / num
except
(ZeroDivisionError, ValueError):
print("Error: Either division by zero
or invalid input occurred.")
```
### Raising
Exceptions Manually
You can use
the `raise` keyword to raise an exception intentionally:
```python
def
check_age(age):
if age < 0:
raise ValueError("Age cannot be
negative.")
return age
try:
age = check_age(-1)
except
ValueError as e:
print(e)
```
This raises
a `ValueError` if the age is negative, and it’s caught and handled in the
`except` block.
### Example:
Using `try-except` with Files
```python
try:
file = open("example.txt",
"r")
content = file.read()
print(content)
except
FileNotFoundError:
print("The file does not exist.")
except
IOError:
print("An IOError occurred while
reading the file.")
finally:
file.close() # Ensures the file is closed
```
### Best
Practices with `try-except`
1. **Catch
specific exceptions**: Avoid using a general `except` block unless absolutely
necessary. It’s better to handle specific exceptions so you can better
understand what went wrong.
2. **Use
`finally` to clean up resources**: Always close files, database connections, or
release any other resources in the `finally` block.
3. **Handle
exceptions gracefully**: Ensure that the program handles errors in a way that
doesn’t confuse the user or cause unexpected behavior.
4. **Do not
overuse `try-except`**: Use exception handling only where necessary. Avoid
placing large blocks of code inside a `try` block—focus on smaller sections
where an error is likely to occur.