Forgot bit locker pin, forgot bit locker recovery key, 5 Easy ways to fix
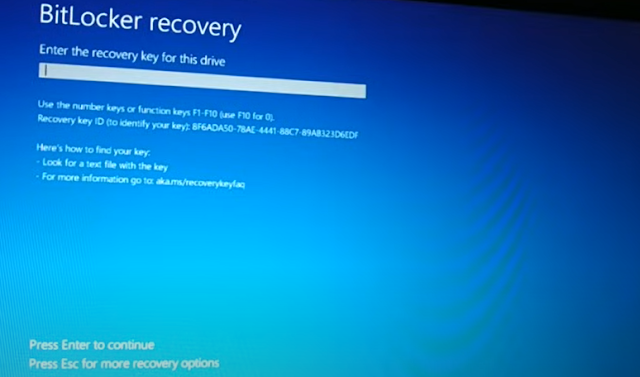
In JavaScript, an array is a special type of object used to store multiple values in a single variable. Arrays are ordered collections of elements, which can be of any type, including numbers, strings, objects, and even other arrays. Each element in an array has an index, which starts at 0.
### Creating Arrays
There are several ways to create arrays in JavaScript:
1. **Using Array Literals**:
```javascript
let fruits =
["Apple", "Banana", "Cherry"];
```
2. **Using the `Array` Constructor**:
```javascript
let numbers = new
Array(1, 2, 3, 4, 5);
```
3. **Using the `Array.of` Method**:
```javascript
let mixedArray =
Array.of(1, 'two', { three: 3 });
```
### Accessing Array Elements
You can access elements in an array using their index:
```javascript
let fruits = ["Apple", "Banana",
"Cherry"];
console.log(fruits[0]); // "Apple"
console.log(fruits[2]); // "Cherry"
```
### Modifying Array Elements
You can modify elements by accessing their index and
assigning a new value:
```javascript
fruits[1] = "Blueberry";
console.log(fruits); // ["Apple", "Blueberry",
"Cherry"]
```
### Array Properties and Methods
Arrays come with a variety of properties and methods that
allow you to manipulate them:
#### `length` Property
The `length` property returns the number of elements in the
array:
```javascript
console.log(fruits.length); // 3
```
#### Common Methods
1. **`push`**: Adds one or more elements to the end of an
array.
```javascript
fruits.push("Date");
console.log(fruits);
// ["Apple", "Blueberry", "Cherry",
"Date"]
```
2. **`pop`**: Removes the last element from an array and
returns that element.
```javascript
let lastFruit =
fruits.pop();
console.log(lastFruit); // "Date"
console.log(fruits);
// ["Apple", "Blueberry", "Cherry"]
```
3. **`shift`**: Removes the first element from an array and
returns that element.
```javascript
let firstFruit =
fruits.shift();
console.log(firstFruit); // "Apple"
console.log(fruits);
// ["Blueberry", "Cherry"]
```
4. **`unshift`**: Adds one or more elements to the beginning
of an array.
```javascript
fruits.unshift("Apricot");
console.log(fruits);
// ["Apricot", "Blueberry", "Cherry"]
```
5. **`indexOf`**: Returns the first index at which a given
element can be found, or -1 if it is not present.
```javascript
let index =
fruits.indexOf("Cherry");
console.log(index);
// 2
```
6. **`splice`**: Adds or removes elements from an array.
```javascript
// Removing elements
fruits.splice(1, 1);
// Removes 1 element at index 1
console.log(fruits);
// ["Apricot", "Cherry"]
// Adding elements
fruits.splice(1, 0,
"Banana");
console.log(fruits);
// ["Apricot", "Banana", "Cherry"]
```
7. **`slice`**: Returns a shallow copy of a portion of an
array into a new array.
```javascript
let newFruits = fruits.slice(1,
3);
console.log(newFruits); // ["Banana", "Cherry"]
```
8. **`concat`**: Merges two or more arrays.
```javascript
let moreFruits =
["Date", "Elderberry"];
let allFruits =
fruits.concat(moreFruits);
console.log(allFruits); // ["Apricot", "Banana",
"Cherry", "Date", "Elderberry"]
```
9. **`forEach`**: Executes a provided function once for each
array element.
```javascript
fruits.forEach(fruit
=> console.log(fruit));
// Output:
//
"Apricot"
//
"Banana"
// "Cherry"
```
10. **`map`**: Creates a new array with the results of
calling a provided function on every element in the calling array.
```javascript
let upperCaseFruits
= fruits.map(fruit => fruit.toUpperCase());
console.log(upperCaseFruits); // ["APRICOT",
"BANANA", "CHERRY"]
```
11. **`filter`**: Creates a new array with all elements that
pass the test implemented by the provided function.
```javascript
let longNamedFruits
= fruits.filter(fruit => fruit.length > 5);
console.log(longNamedFruits);
// ["Apricot", "Banana"]
```
12. **`reduce`**: Executes a reducer function on each element
of the array, resulting in a single output value.
```javascript
let totalLength =
fruits.reduce((total, fruit) => total + fruit.length, 0);
console.log(totalLength); // 18
```
13. **`find`**: Returns the value of the first element in the
array that satisfies the provided testing function.
```javascript
let foundFruit =
fruits.find(fruit => fruit.startsWith("B"));
console.log(foundFruit);
// "Banana"
```
14. **`findIndex`**: Returns the index of the first element
in the array that satisfies the provided testing function.
```javascript
let foundIndex =
fruits.findIndex(fruit => fruit.startsWith("B"));
console.log(foundIndex);
// 1
```
### Multidimensional Arrays
Arrays can also contain other arrays, creating
multidimensional arrays:
```javascript
let matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
console.log(matrix[0][1]); // 2
```
### Example Usage
Here is a complete example demonstrating various array
operations:
```javascript
let animals = ["Cat", "Dog",
"Elephant"];
console.log(animals.length); // 3
animals.push("Fox");
console.log(animals); // ["Cat", "Dog",
"Elephant", "Fox"]
animals.pop();
console.log(animals); // ["Cat", "Dog",
"Elephant"]
animals.shift();
console.log(animals); // ["Dog",
"Elephant"]
animals.unshift("Bear");
console.log(animals); // ["Bear", "Dog",
"Elephant"]
let dogIndex = animals.indexOf("Dog");
console.log(dogIndex); // 1
animals.splice(1, 1, "Lion");
console.log(animals); // ["Bear", "Lion",
"Elephant"]
let selectedAnimals = animals.slice(0, 2);
console.log(selectedAnimals); // ["Bear",
"Lion"]
let moreAnimals = ["Giraffe", "Hippo"];
let allAnimals = animals.concat(moreAnimals);
console.log(allAnimals); // ["Bear",
"Lion", "Elephant", "Giraffe", "Hippo"]
allAnimals.forEach(animal => console.log(animal));
// Output:
// "Bear"
// "Lion"
// "Elephant"
// "Giraffe"
// "Hippo"
let upperCaseAnimals = allAnimals.map(animal =>
animal.toUpperCase());
console.log(upperCaseAnimals); // ["BEAR",
"LION", "ELEPHANT", "GIRAFFE", "HIPPO"]
let longNamedAnimals = allAnimals.filter(animal =>
animal.length > 4);
console.log(longNamedAnimals); // ["Elephant",
"Giraffe", "Hippo"]
let totalNameLength = allAnimals.reduce((total, animal) =>
total + animal.length, 0);
console.log(totalNameLength); // 30
let foundAnimal = allAnimals.find(animal =>
animal.startsWith("G"));
console.log(foundAnimal); // "Giraffe"
let foundAnimalIndex = allAnimals.findIndex(animal =>
animal.startsWith("G"));
console.log(foundAnimalIndex); // 3
```
### Summary
- **Arrays**: Ordered collections of elements, which can be
of any type.
- **Creating Arrays**: Using array literals, `Array`
constructor, or `Array.of`.
- **Accessing/Modifying**: Use indices to access or modify
elements.
- **Properties/Methods**: Use various properties (`length`)
and methods (`push`, `pop`, `shift`, `unshift`, etc.) to manipulate arrays.
- **Iteration**: Use loops (`forEach`, `map`, `filter`, etc.)
to iterate over arrays.
- **Multidimensional Arrays**: Arrays can contain other
arrays.
Arrays are a fundamental part of JavaScript and are essential
for managing collections of data.