Forgot bit locker pin, forgot bit locker recovery key, 5 Easy ways to fix
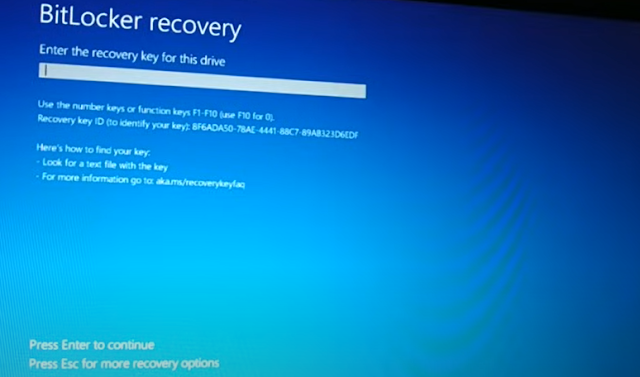
In JavaScript, a `Map` is a built-in object that allows you to store key-value pairs and provides several methods to work with these pairs. Unlike regular objects, where keys are always strings or symbols, `Map` keys can be of any data type, including objects, functions, and primitive values.
### Creating a Map
You can create a new `Map` using the `Map` constructor:
```javascript
let map = new Map();
```
### Adding Key-Value Pairs
Use the `set` method to add key-value pairs to the map:
```javascript
map.set('name', 'Alice');
map.set('age', 30);
map.set('job', 'Engineer');
```
### Accessing Values
Use the `get` method to retrieve the value associated with a
specific key:
```javascript
console.log(map.get('name')); // "Alice"
console.log(map.get('age')); // 30
```
### Checking for Keys
Use the `has` method to check if a map contains a specific
key:
```javascript
console.log(map.has('name')); // true
console.log(map.has('salary')); // false
```
### Removing Key-Value Pairs
Use the `delete` method to remove a key-value pair from the
map:
```javascript
map.delete('job');
console.log(map.has('job')); // false
```
### Getting the Size of a Map
Use the `size` property to get the number of key-value pairs
in the map:
```javascript
console.log(map.size); // 2
```
### Clearing the Map
Use the `clear` method to remove all key-value pairs from the
map:
```javascript
map.clear();
console.log(map.size); // 0
```
### Iterating Over a Map
You can iterate over the key-value pairs in a map using
various methods:
1. **Using `for...of` Loop**:
```javascript
let map = new Map();
map.set('name',
'Alice');
map.set('age', 30);
for (let [key,
value] of map) {
console.log(`${key}: ${value}`);
}
// Output:
// "name:
Alice"
// "age:
30"
```
2. **Using `forEach` Method**:
```javascript
map.forEach((value,
key) => {
console.log(`${key}: ${value}`);
});
// Output:
// "name:
Alice"
// "age:
30"
```
3. **Using Map Iterators**:
- **keys()**:
Returns an iterator of keys.
```javascript
for (let key of map.keys()) {
console.log(key);
}
// Output:
//
"name"
// "age"
```
- **values()**:
Returns an iterator of values.
```javascript
for (let value of
map.values()) {
console.log(value);
}
// Output:
//
"Alice"
// "30"
```
- **entries()**:
Returns an iterator of key-value pairs.
```javascript
for (let [key,
value] of map.entries()) {
console.log(`${key}: ${value}`);
}
// Output:
// "name: Alice"
// "age:
30"
```
### Example Usage
Here is a complete example demonstrating various operations
on a `Map`:
```javascript
// Creating a new Map
let userMap = new Map();
// Adding key-value pairs
userMap.set('username', 'john_doe');
userMap.set('email', 'john@example.com');
userMap.set('age', 25);
// Accessing values
console.log(userMap.get('username')); // "john_doe"
console.log(userMap.get('email')); //
"john@example.com"
// Checking for keys
console.log(userMap.has('age')); // true
console.log(userMap.has('address')); // false
// Removing a key-value pair
userMap.delete('age');
console.log(userMap.has('age')); // false
// Getting the size of the map
console.log(userMap.size); // 2
// Iterating over the map
userMap.forEach((value, key) => {
console.log(`${key}: ${value}`);
});
// Output:
// "username: john_doe"
// "email: john@example.com"
// Clearing the map
userMap.clear();
console.log(userMap.size); // 0
```
### Summary
- A `Map` is an object that holds key-value pairs, where keys
can be of any data type.
- Use `set` to add key-value pairs and `get` to retrieve
values.
- Use `has` to check for the presence of a key and `delete`
to remove key-value pairs.
- Use `size` to get the number of key-value pairs and `clear`
to remove all pairs.
- Iterate over a `Map` using `for...of`, `forEach`, or the
map's iterator methods (`keys()`, `values()`, `entries()`).
Maps provide a flexible and efficient way to manage
collections of key-value pairs, offering more features and capabilities than
regular objects for this purpose.