Forgot bit locker pin, forgot bit locker recovery key, 5 Easy ways to fix
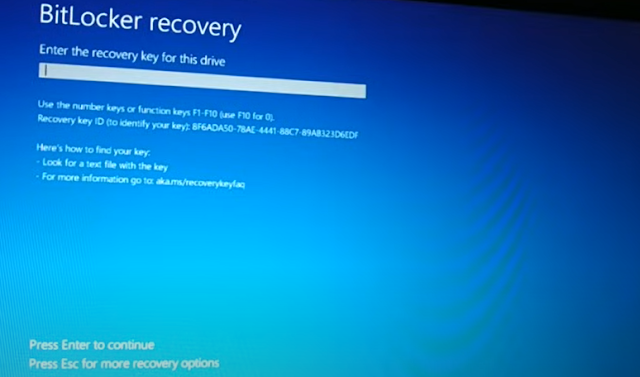
A **`while` loop** in Python is used to repeatedly execute a block of code as long as a given condition is `True`. It's commonly used when the number of iterations is not known ahead of time and depends on some dynamic condition.
### Syntax
of `while` loop:
```python
while condition:
# code block
```
-
**`condition`**: This is an expression that gets evaluated before each
iteration of the loop. If the condition is `True`, the loop continues; if it's
`False`, the loop stops.
- The code
inside the loop (called the **loop body**) will continue to execute until the
condition becomes `False`.
### Example
of a `while` loop:
```python
# Example:
Print numbers from 1 to 5
count = 1
while count
<= 5:
print(count)
count += 1
# increment the count
```
**Output:**
```
1
2
3
4
5
```
In this
example:
- The loop
runs as long as `count <= 5`.
- Each time
through the loop, `count` is incremented by 1.
- The loop
stops when `count` becomes 6 (because `6 <= 5` is `False`).
### `while`
with `else`:
A `while`
loop can also have an optional `else` block, which is executed when the
condition becomes `False`.
```python
count = 1
while count
<= 3:
print(count)
count += 1
else:
print("Loop finished")
```
**Output:**
```
1
2
3
Loop
finished
```
### Infinite
Loop:
If the condition
never becomes `False`, the loop will run indefinitely. This is called an
**infinite loop**.
```python
# Be
careful, this is an infinite loop:
while True:
print("This will run forever!")
```
### Breaking
out of a `while` loop:
You can use
the `break` statement to exit the loop prematurely, regardless of the
condition.
```python
count = 1
while count
<= 5:
if count == 3:
break
# Exit the loop when count equals 3
print(count)
count += 1
```
**Output:**
```
1
2
```
### Skipping
an iteration:
You can use
the `continue` statement to skip the rest of the code in the current iteration and
jump to the next iteration.
```python
count = 0
while count
< 5:
count += 1
if count == 3:
continue # Skip the rest of the code when count equals
3
print(count)
```
**Output:**
```
1
2
4
5
```
Here, when
`count` is 3, the `continue` statement skips the `print(count)` statement and
moves to the next iteration.
### Summary:
- `while`
loops are used when you want to repeat a block of code as long as a condition
is `True`.