Forgot bit locker pin, forgot bit locker recovery key, 5 Easy ways to fix
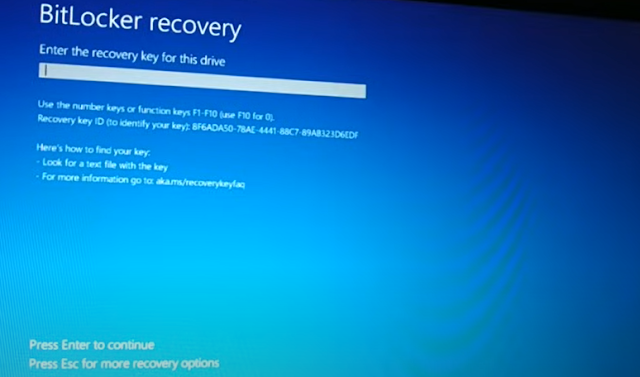
In Python, **scope** refers to the region or context in a program where a variable or function is accessible. The scope determines the visibility and lifetime of a variable. Understanding scope helps you manage where variables are accessible and how they interact across different parts of your program.
### Types of
Scopes in Python
There are
four types of scopes in Python:
1. **Local
Scope**
2.
**Enclosing Scope**
3. **Global
Scope**
4.
**Built-in Scope**
These scopes
follow the **LEGB Rule**, which is the order in which Python resolves the scope
of variables: **Local, Enclosing, Global, Built-in**.
### 1.
**Local Scope**
Variables
defined inside a function or a block are in the local scope and can only be
accessed within that function or block.
####
Example:
```python
def my_function():
x = 10
# Local variable
print(x)
# Accessible within the function
my_function() # Output: 10
print(x) # Raises NameError because x is not
accessible outside the function
```
### 2.
**Enclosing Scope (Nonlocal)**
This refers
to the scope of outer functions in case of nested functions. Variables in the
enclosing scope can be accessed by inner functions using the `nonlocal`
keyword.
####
Example:
```python
def
outer_function():
x = 5
# Enclosing scope
def inner_function():
nonlocal x
x = 10
# Modifies the variable in the enclosing scope
print(f"Inner x: {x}")
inner_function()
print(f"Outer x: {x}")
outer_function()
# Output:
# Inner x:
10
# Outer x:
10
```
In the
example, `nonlocal x` modifies the variable `x` in the enclosing scope
(`outer_function`).
### 3.
**Global Scope**
Variables
defined outside of all functions or blocks are in the global scope. They can be
accessed from anywhere in the program, including inside functions. To modify a
global variable inside a function, the `global` keyword must be used.
####
Example:
```python
x = 20 # Global variable
def
my_function():
global x
x = 30
# Modifies the global variable
print(f"Inside function x: {x}")
my_function() # Output: Inside function x: 30
print(f"Outside
function x: {x}") # Output: Outside
function x: 30
```
Without the
`global` keyword, `x` inside the function would be treated as a local variable,
separate from the global `x`.
### 4.
**Built-in Scope**
This is the
outermost scope that contains all the names that are built into Python. These
are functions and exceptions like `print()`, `len()`, `range()`, `str()`, and
`Exception`.
####
Example:
```python
# len() is a
built-in function
my_list =
[1, 2, 3, 4]
print(len(my_list)) # Output: 4
```
Python will
first check the local, enclosing, and global scopes for a variable name. If it
is not found, it will look into the built-in scope.
### **The
LEGB Rule**
The **LEGB
rule** defines how Python resolves variable names:
1. **Local**:
Python first looks in the local scope (inside the current function).
2.
**Enclosing**: If it doesn’t find it locally, it looks in the enclosing
function’s scope (in case of nested functions).
3.
**Global**: Then, it checks the global scope (outside all functions).
4.
**Built-in**: If it still doesn’t find it, it checks the built-in scope.
#### Example
of LEGB:
```python
x =
"global"
def outer():
x = "enclosing"
def inner():
x = "local"
print(x) # Looks for 'x' in the local scope first
inner()
outer() # Output: local
```
Python
resolves `x` using the LEGB rule:
- `x` is
found in the **local scope** of `inner()`, so it prints `"local"`.
### **Global
vs. Local Variables**
By default,
variables created inside a function are local and only available within that
function. Variables defined outside a function are global and can be accessed
from anywhere. To modify global variables inside functions, you must declare them
with the `global` keyword.
####
Example:
```python
x = 50 # Global variable
def
change_value():
x = 100
# This creates a new local variable, doesn't change global x
print(f"Inside function x: {x}")
change_value() # Output: Inside function x: 100
print(f"Outside
function x: {x}") # Output: Outside
function x: 50
```
### **Using
the `global` Keyword**
The `global`
keyword allows you to modify a global variable from within a function.
####
Example:
```python
x = 50 # Global variable
def
change_value():
global x
x = 100
# Modify the global x
print(f"Inside function x: {x}")
change_value() # Output: Inside function x: 100
print(f"Outside
function x: {x}") # Output: Outside
function x: 100
```
### **Using
the `nonlocal` Keyword**
The
`nonlocal` keyword is used to modify variables in the enclosing scope (outside
the local scope but not global). It is useful in nested functions.
####
Example:
```python
def
outer_function():
x = 10
# Enclosing scope
def inner_function():
nonlocal x
x = 20
# Modify the enclosing x
print(f"Inner x: {x}")
inner_function()
print(f"Outer x: {x}")
outer_function()
# Output:
# Inner x:
20
# Outer x:
20
```
Without
`nonlocal`, `x` inside `inner_function()` would create a new local variable
instead of modifying the `x` from the enclosing scope.
### Best
Practices:
1. **Avoid
Global Variables**: Global variables can lead to code that is difficult to
maintain and debug. Use local variables whenever possible.
2. **Use
`global` and `nonlocal` Sparingly**: These keywords can make code harder to
follow. Use them only when necessary.
3.
**Understand the LEGB Rule**: Familiarize yourself with how Python resolves
variable names, as it helps avoid scope-related issues.
### Summary:
- **Scope**
determines where variables are accessible in your code.
- **Local
scope**: Variables declared inside a function or block.
-
**Enclosing scope**: Variables from outer functions in case of nested
functions.
- **Global
scope**: Variables declared outside of all functions.
- **Built-in
scope**: Python’s built-in names like `len()` and `print()`.
- The **LEGB
rule** is used by Python to resolve the scope of variables: **Local → Enclosing
→ Global → Built-in**.
Understanding
Python’s scope rules helps in writing clean, efficient, and bug-free code by
properly managing where and how variables are accessed and modified.