Forgot bit locker pin, forgot bit locker recovery key, 5 Easy ways to fix
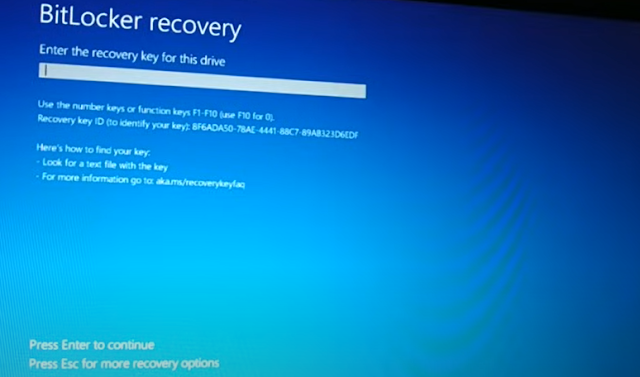
**Polymorphism** in Python is a core concept of **Object-Oriented Programming (OOP)** that allows objects of different classes to be treated as objects of a common superclass. It refers to the ability of different objects to respond to the same method in their own way. Polymorphism promotes flexibility and makes code more dynamic and reusable.
### Key
Concepts:
1. **Method
Polymorphism**: Different classes can define methods with the same name, and
the method will behave according to the class it is called from.
2.
**Polymorphism with Inheritance**: Classes that inherit from a common parent
class can override the methods of the parent class. The method behavior depends
on the object that calls it.
### 1.
**Polymorphism in Built-in Functions**
Many Python
functions exhibit polymorphism by accepting objects of different types. For
example, the `len()` function works for different data types like strings,
lists, or tuples.
####
Example:
```python
print(len("Hello")) # Output: 5 (Length of a string)
print(len([1,
2, 3, 4])) # Output: 4 (Length of a
list)
print(len((1,
2, 3))) # Output: 3 (Length of a
tuple)
```
Here, the
`len()` function works for different data types because it expects any object
that implements the `__len__()` method, demonstrating polymorphism.
### 2. **Polymorphism
with Functions**
A single
function can work with objects of different classes as long as those objects
share a common method.
####
Example:
```python
class Dog:
def speak(self):
return "Bark"
class Cat:
def speak(self):
return "Meow"
def
animal_sound(animal):
print(animal.speak())
# Creating
instances of different classes
dog = Dog()
cat = Cat()
# Passing
objects to a function
animal_sound(dog) # Output: Bark
animal_sound(cat) # Output: Meow
```
In this
example, the `animal_sound()` function takes any object that has the `speak()`
method, and it behaves differently depending on whether the object is a `Dog`
or a `Cat`.
### 3. **Polymorphism
in Class Methods**
Polymorphism
allows the same method to be used for different object types, even when the
method is defined in different classes.
####
Example:
```python
class
Rectangle:
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
class
Circle:
def __init__(self, radius):
self.radius = radius
def area(self):
return 3.14 * self.radius * self.radius
# Creating
objects of different classes
rect =
Rectangle(10, 20)
circ =
Circle(7)
# Using the
same method name 'area()' on different objects
print(rect.area()) # Output: 200
print(circ.area()) # Output: 153.86
```
Here, both
`Rectangle` and `Circle` have an `area()` method, but the behavior is different
based on the object type. This is an example of polymorphism in action.
### 4. **Polymorphism
with Inheritance**
Polymorphism
is often used in conjunction with inheritance, where a parent class defines a
method, and child classes override the method. You can treat objects of child
classes as objects of the parent class, and the correct method will be called according
to the object's type.
####
Example:
```python
# Parent
class
class
Animal:
def speak(self):
return "Some sound"
# Child
class
class Dog(Animal):
def speak(self):
return "Bark"
# Another
Child class
class
Cat(Animal):
def speak(self):
return "Meow"
# A function
that works on the parent class
def
animal_speak(animal):
print(animal.speak())
#
Polymorphism in action
animals =
[Dog(), Cat()]
for animal
in animals:
animal_speak(animal)
# Output:
# Bark
# Meow
```
In this
example:
- Both `Dog`
and `Cat` inherit from `Animal`, and each class overrides the `speak()` method.
- The
`animal_speak()` function accepts objects of the parent class `Animal`, but
when passed objects of the child classes, the method specific to the child
class is called.
### 5.
**Polymorphism with Abstract Classes and Interfaces**
In Python,
**abstract base classes** (using the `abc` module) enforce that derived classes
implement specific methods, providing a structure for polymorphism.
#### Example
of Abstract Class Polymorphism:
```python
from abc
import ABC, abstractmethod
# Abstract
base class
class
Animal(ABC):
@abstractmethod
def speak(self):
pass
# Derived
class Dog
class
Dog(Animal):
def speak(self):
return "Bark"
# Derived
class Cat
class
Cat(Animal):
def speak(self):
return "Meow"
# Function
using polymorphism
def
animal_sound(animal):
print(animal.speak())
# Creating
instances
dog = Dog()
cat = Cat()
# Using the
function
animal_sound(dog) # Output: Bark
animal_sound(cat) # Output: Meow
```
Here, the
`Animal` class is abstract and ensures that any subclass must implement the
`speak()` method. This promotes polymorphism because the `animal_sound()`
function can work with any object that follows the `Animal` interface.
### 6.
**Polymorphism with Operator Overloading**
Polymorphism
also exists in Python through **operator overloading**, where the same operator
behaves differently depending on the operands' types.
####
Example:
```python
# Using '+'
operator on integers
print(10 +
5) # Output: 15
# Using '+'
operator on strings
print("Hello"
+ " World") # Output: Hello
World
```
Here, the
`+` operator behaves differently depending on whether it's applied to integers
or strings, demonstrating polymorphism through operator overloading.
### Benefits
of Polymorphism:
1. **Code
Flexibility**: You can write more generic code that works with different types
of objects.
2.
**Reusability**: Polymorphism allows you to reuse the same interface for
different underlying forms (objects), reducing the need to rewrite code.
3.
**Extensibility**: You can add new types of objects without changing the polymorphic
function, making it easy to extend your application.
### Summary:
-
**Polymorphism** allows the same method or function to behave differently
depending on the object it is called on.
- It works
with both functions and methods, enabling dynamic behavior based on object
types.
-
Polymorphism is especially useful when combined with **inheritance**, allowing
for method overriding and promoting flexible and reusable code.
- **Abstract
base classes** enforce polymorphism by defining methods that must be implemented
in child classes.
Polymorphism
helps to write cleaner, more modular, and maintainable code, especially in
scenarios involving multiple types of objects that share common interfaces.