Forgot bit locker pin, forgot bit locker recovery key, 5 Easy ways to fix
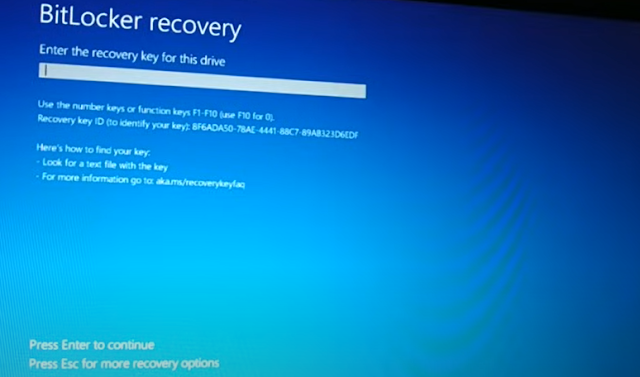
A **Python Lambda function** is a small anonymous function defined with the keyword `lambda`. Unlike normal functions defined using `def`, a lambda function is used for simple, one-liner functions, and doesn't require a name. It can take any number of arguments but can only have one expression.
### Syntax
of a Lambda Function:
```python
lambda arguments:
expression
```
-
**arguments**: Parameters to the function
-
**expression**: A single expression that the function will return
### How to
Use Lambda Functions:
1. **Basic
Example**:
Here's a lambda function that adds 2 to a
number.
```python
add_two = lambda x: x + 2
print(add_two(5)) # Output: 7
```
2.
**Multiple Arguments**:
You can have multiple arguments in a lambda
function.
```python
multiply = lambda a, b: a * b
print(multiply(4, 3)) # Output: 12
```
3. **Use in
Higher-Order Functions**:
Lambda functions are often used with
functions like `map()`, `filter()`, and `reduce()`.
- **Using with `map()`**:
```python
numbers = [1, 2, 3, 4]
doubled = list(map(lambda x: x * 2,
numbers))
print(doubled) # Output: [2, 4, 6, 8]
```
- **Using with `filter()`**:
```python
numbers = [1, 2, 3, 4, 5]
even = list(filter(lambda x: x % 2 == 0,
numbers))
print(even) # Output: [2, 4]
```
- **Using with `reduce()`** (from the
`functools` module):
```python
from functools import reduce
numbers = [1, 2, 3, 4]
product = reduce(lambda x, y: x * y,
numbers)
print(product) # Output: 24
```
### When to
Use Lambda Functions:
- When you
need a small function for a short period of time.
- Useful
when passing simple functionality to higher-order functions.
- Avoid
using lambda for complex logic—use `def` for readability and maintainability.
###
Limitations:
- Lambdas are
limited to a single expression. They cannot contain multiple statements.
- For more
complex functions, it’s better to define a regular function using `def`.