Forgot bit locker pin, forgot bit locker recovery key, 5 Easy ways to fix
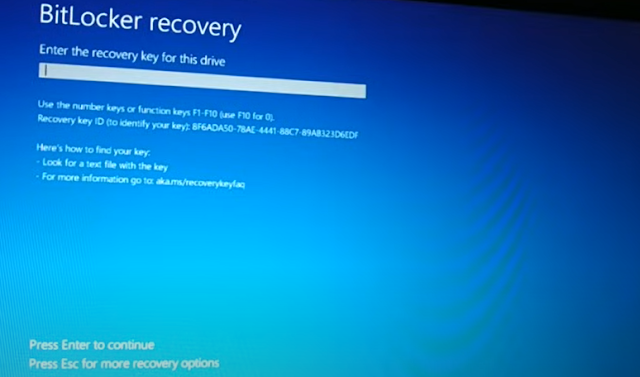
In Python, an **iterator** is an object that allows you to traverse (or iterate) through all the elements of a collection (like a list, tuple, or dictionary) one element at a time. Iterators are an essential part of **iterable objects** such as sequences, which include strings, lists, tuples, and other containers.
### Key
Concepts:
-
**Iterable**: An object capable of returning its elements one at a time.
Examples include lists, tuples, strings, dictionaries, etc. Any object that can
be looped over with a `for` loop is iterable.
-
**Iterator**: An object that represents a stream of data. It returns the data
one element at a time when called with the `next()` function.
### 1. **How
Iterators Work**
-
**`__iter__()`**: This method returns the iterator object itself.
-
**`__next__()`**: This method returns the next value from the iterator. When
there are no more items to return, it raises a `StopIteration` exception.
### 2. **Using
an Iterator**
To use an
iterator, you need an **iterable object** (like a list, tuple, etc.) and you
convert it to an iterator using the `iter()` function. You can then retrieve
elements one by one using `next()`.
####
Example:
```python
# List is an
iterable
my_list =
[1, 2, 3]
# Get an
iterator object using iter()
my_iter =
iter(my_list)
# Use next()
to access elements one by one
print(next(my_iter)) # Output: 1
print(next(my_iter)) # Output: 2
print(next(my_iter)) # Output: 3
# After the
last element, next() raises StopIteration
#
print(next(my_iter)) # Raises
StopIteration
```
### 3. **Looping
through an Iterator**
You
typically don’t need to call `next()` manually, since iterators are
automatically handled by looping constructs like `for` loops.
#### Example
with `for` loop:
```python
my_list =
[1, 2, 3]
for item in
my_list:
print(item)
# Output:
# 1
# 2
# 3
```
### 4.
**Creating a Custom Iterator**
You can
create your own custom iterators by defining a class with the `__iter__()` and
`__next__()` methods.
#### Example
of a Custom Iterator:
```python
class
Counter:
def __init__(self, low, high):
self.current = low
self.high = high
def __iter__(self):
return self
def __next__(self):
if self.current > self.high:
raise StopIteration
else:
self.current += 1
return self.current - 1
# Create an
instance of Counter
counter =
Counter(1, 5)
# Use the
iterator
for num in
counter:
print(num)
# Output:
# 1
# 2
# 3
# 4
# 5
```
### 5. **Python
Generators as Iterators**
A
**generator** is a simpler way to create iterators. Instead of defining
`__iter__()` and `__next__()` methods, you can use the `yield` keyword in a
function to turn it into a generator. Every time `yield` is encountered, the
function returns a value and pauses its state. The next time the generator is
called, it resumes from where it left off.
#### Example
of a Generator:
```python
def
count_up_to(max):
count = 1
while count <= max:
yield count
count += 1
# Create a
generator object
counter =
count_up_to(5)
# Iterate
over the generator
for num in
counter:
print(num)
# Output:
# 1
# 2
# 3
# 4
# 5
```
### 6.
**Common Uses of Iterators**
- **File
Iteration**: Reading files line by line is a common use of iterators. Files in
Python are inherently iterable.
```python
with open('file.txt') as f:
for line in f:
print(line)
```
-
**Efficient Data Processing**: Iterators allow you to process large datasets
lazily (on demand), making them memory-efficient.
### 7.
**Difference between Iterators and Iterables**
-
**Iterable**: An object capable of returning its members one by one. It must
implement the `__iter__()` method, which returns an iterator.
-
**Iterator**: An object with a state that remembers where it is during
iteration. It implements the `__next__()` method to return the next value and
raises `StopIteration` when there are no more values.
####
Example:
```python
# List is an
iterable
my_list =
[1, 2, 3]
# Using iter()
returns an iterator
my_iter =
iter(my_list)
# Iterator
object supports next()
print(next(my_iter)) # Output: 1
```
### 8.
**Built-in Functions for Iterators**
-
**`iter()`**: Converts an iterable into an iterator.
-
**`next()`**: Retrieves the next item from an iterator.
-
**`zip()`**: Combines multiple iterables into a single iterator.
-
**`enumerate()`**: Provides an iterator that returns both the index and value
of elements in an iterable.
-
**`map()`**: Applies a function to all elements in an iterable and returns an
iterator.
### Summary:
- An
**iterator** is an object that allows sequential traversal of data (e.g.,
elements in a list).
- Use
`iter()` to get an iterator from an iterable and `next()` to get the next
element.
- Custom
iterators can be created using the `__iter__()` and `__next__()` methods.
-
**Generators** simplify iterator creation using the `yield` keyword.
- Iterators
are especially useful for memory-efficient data processing and lazy evaluation.
Iterators
and generators are widely used in Python for looping over collections,
processing data streams, and handling large datasets efficiently.