Forgot bit locker pin, forgot bit locker recovery key, 5 Easy ways to fix
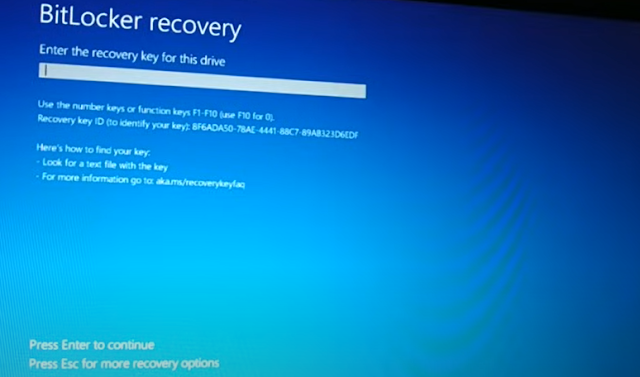
In Python, the `if...else` statement is a control flow structure that allows you to execute certain blocks of code based on specific conditions. It's used to make decisions in your code, where different actions are taken depending on whether a condition is `True` or `False`.
### Basic Structure
The basic structure of an `if...else` statement is:
```python
if
condition:
# code to execute if the condition is True
else:
# code to execute if the condition is False
```
### How It
Works
- **`if`
statement**: The `if` keyword is followed by a condition (an expression that
evaluates to `True` or `False`). If the condition is `True`, the code block
inside the `if` statement is executed.
- **`else`
statement**: The `else` keyword follows the `if` block. If the `if` condition
is `False`, the code block inside the `else` statement is executed.
### Example
```python
age = 18
if age >=
18:
print("You are an adult.")
else:
print("You are not an adult.")
```
**Output:**
```
You are an
adult.
```
In this
example, the condition `age >= 18` evaluates to `True`, so the first block
of code (inside the `if` statement) is executed.
### The
`elif` Clause
The `elif`
(short for "else if") keyword allows you to check multiple conditions
in sequence. It's used when you want to test additional conditions if the
previous `if` or `elif` conditions are `False`.
```python
score = 85
if score
>= 90:
print("Grade: A")
elif score
>= 80:
print("Grade: B")
elif score
>= 70:
print("Grade: C")
else:
print("Grade: F")
```
**Output:**
```
Grade: B
```
Here, the
first condition `score >= 90` is `False`, so Python moves to the next `elif`
statement. The condition `score >= 80` is `True`, so the code inside that
block is executed.
### Nested
`if` Statements
You can nest
`if...else` statements inside each other to create more complex decision
structures.
```python
number = 15
if number
> 0:
print("The number is positive.")
if number % 2 == 0:
print("It is an even number.")
else:
print("It is an odd number.")
else:
print("The number is not
positive.")
```
**Output:**
```
The number
is positive.
It is an odd
number.
```
In this example, the first `if` statement checks if the number is positive. If it is, the nested `if...else` statement checks whether the number is even or odd.
### Using
`if...else` with Comparison and Logical Operators
You can use
comparison operators (`==`, `!=`, `<`, `>`, `<=`, `>=`) and logical
operators (`and`, `or`, `not`) within `if...else` conditions to create more
complex logical tests.
```python
age = 20
has_id =
True
if age >=
18 and has_id:
print("You are allowed to
enter.")
else:
print("You are not allowed to
enter.")
```
**Output:**
```
You are
allowed to enter.
```
In this
case, the condition checks if both `age >= 18` and `has_id` are `True`.
Since both are `True`, the first block of code is executed.
### The
`else if` Ladder
When you
have multiple conditions to check, you can chain multiple `elif` clauses
together. Python will evaluate each condition in sequence, and the first one
that is `True` will have its corresponding block of code executed. If none of
the conditions are `True`, the `else` block is executed.
```python
temperature
= 75
if
temperature > 85:
print("It's hot outside.")
elif
temperature > 60:
print("The weather is nice.")
elif
temperature > 32:
print("It's getting chilly.")
else:
print("It's freezing!")
```
**Output:**
```
The weather
is nice.
```
### Ternary
(Conditional) Operator
Python also
supports a shorter form of the `if...else` statement called the ternary
operator. It's useful when you want to assign a value to a variable based on a
condition.
```python
# Syntax:
value_if_true if condition else value_if_false
age = 17
status =
"Adult" if age >= 18 else "Minor"
print(status) # Output: Minor
```
###
Conclusion
The
`if...else` statement is a fundamental building block for decision-making in
Python programs. It allows your code to react differently based on varying
conditions, enabling more complex and dynamic behavior. Understanding how to
use `if`, `elif`, and `else` effectively is essential for writing logical and
robust Python programs.