Forgot bit locker pin, forgot bit locker recovery key, 5 Easy ways to fix
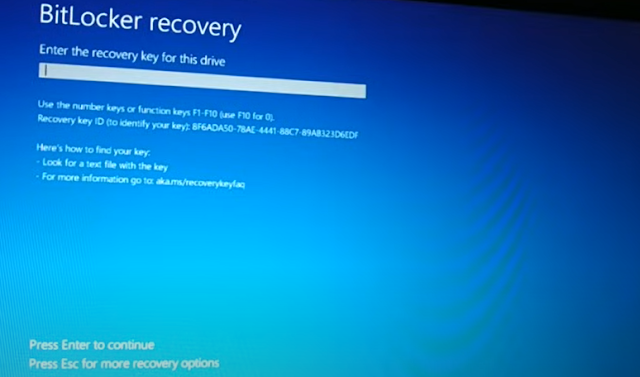
A **`for` loop** in Python is used to iterate over a sequence (like a list, tuple, string, dictionary, or range) and execute a block of code for each item in that sequence. It's ideal when you know the number of iterations beforehand or when working with iterable objects.
### Syntax of a `for` loop:
```python
for variable in sequence:
# code block
```
- **`variable`**: This is a temporary variable that takes the value of each element in the sequence during each iteration.
-
**`sequence`**: This can be any iterable like a list, tuple, string,
dictionary, or range of numbers.
### Example
of a `for` loop:
```python
# Example:
Iterating through a list
numbers =
[1, 2, 3, 4, 5]
for num in
numbers:
print(num)
```
**Output:**
```
1
2
3
4
5
```
In this
example:
- The loop
iterates through the list `numbers`.
- During each
iteration, the value of `num` takes one item from the list, and the `print()`
function outputs it.
###
Iterating over a string:
```python
# Example:
Iterating through a string
for char in
"Python":
print(char)
```
**Output:**
```
P
y
t
h
o
n
```
### Using
`range()` with `for` loop:
The
`range()` function is often used to generate a sequence of numbers, making it
useful in `for` loops for iterating a specific number of times.
```python
# Example:
Using range()
for i in
range(5): # range(5) generates numbers
from 0 to 4
print(i)
```
**Output:**
```
0
1
2
3
4
```
The
`range(start, stop, step)` function allows you to specify:
- `start`:
Starting number (inclusive).
- `stop`:
Ending number (exclusive).
- `step`:
Difference between each number (default is 1).
For example:
```python
for i in
range(2, 10, 2):
print(i)
```
**Output:**
```
2
4
6
8
```
###
Iterating over a dictionary:
When
iterating over a dictionary, you can access both keys and values.
```python
# Example:
Iterating through a dictionary
my_dict =
{'name': 'Alice', 'age': 25, 'city': 'New York'}
for key,
value in my_dict.items():
print(key, value)
```
**Output:**
```
name Alice
age 25
city New
York
```
### Nested
`for` loops:
You can nest
`for` loops to iterate over multi-dimensional data (like lists of lists).
```python
# Example:
Nested for loop
matrix =
[[1, 2, 3], [4, 5, 6], [7, 8, 9]]
for row in
matrix:
for num in row:
print(num, end=' ')
print()
# move to the next line
```
**Output:**
```
1 2 3
4 5 6
7 8 9
```
### `for`
loop with `else`:
Similar to
`while` loops, `for` loops can also have an `else` block, which runs after the
loop completes all iterations unless the loop is exited with a `break`.
```python
for i in
range(3):
print(i)
else:
print("Loop completed")
```
**Output:**
```
0
1
2
Loop
completed
```
### Breaking
out of a `for` loop:
You can use
the `break` statement to exit the loop early, even if the sequence has not been
fully iterated over.
```python
for num in
range(5):
if num == 3:
break
# Exit the loop when num equals 3
print(num)
```
**Output:**
```
0
1
2
```
### Skipping
iterations with `continue`:
You can use
the `continue` statement to skip the current iteration and move to the next
one.
```python
for num in
range(5):
if num == 2:
continue # Skip the rest of the code for num == 2
print(num)
```
**Output:**
```
0
1
3
4
```
### Summary:
- `for`
loops are best used when you need to iterate over a sequence of items like
lists, strings, or ranges.
- The
`range()` function is often used to generate sequences of numbers.
- You can
use `break` to exit a loop early and `continue` to skip an iteration.