Forgot bit locker pin, forgot bit locker recovery key, 5 Easy ways to fix
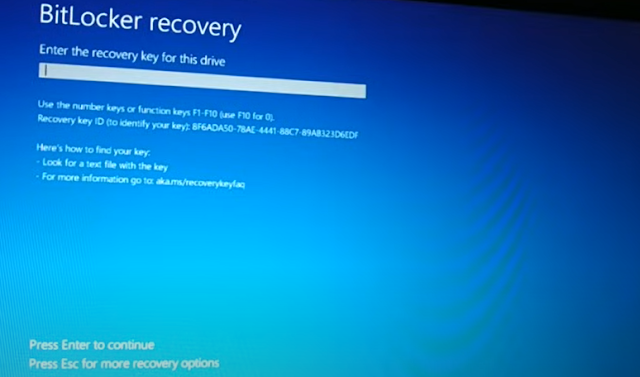
In Python, comments are used to explain code, making it easier to understand, maintain, and debug. Comments are not executed by the Python interpreter, so they do not affect the program's behavior. Here's how to use comments in Python:
### 1.
**Single-Line Comments**
- Single-line comments start with the `#`
symbol. Anything following `#` on that line is considered a comment and is
ignored by the interpreter.
- **Usage**: To explain specific lines of
code or provide brief annotations.
**Example**:
```python
# This is a single-line comment
x = 5
# This assigns the value 5 to the variable x
print(x)
# This will print the value of x
```
- In the above example, the comments explain
what each line of code does.
### 2.
**Multi-Line Comments**
- Python does not have a specific syntax for
multi-line comments, but you can use multiple single-line comments or
triple-quoted strings (`'''` or `"""`) for this purpose.
- **Usage**: To provide detailed
explanations, block descriptions, or to temporarily disable a block of code
during debugging.
**Example 1: Multiple Single-Line
Comments**:
```python
# This is a multi-line comment
# that spans across several lines.
# Each line starts with a hash symbol.
```
**Example 2: Triple-Quoted Strings**:
```python
"""
This is a multi-line comment using
triple-quoted strings. Although intended
for documentation, it can be used as a
comment.
"""
print("Hello, World!")
```
- Triple-quoted strings are often used for
**docstrings** in Python, which are a special type of comment used to describe
functions, classes, and modules.
### 3.
**Docstrings**
- Docstrings are a type of comment used to
document a function, class, or module. They are written using triple quotes
(`'''` or `"""`) and are placed immediately after the function
or class definition.
- **Usage**: To provide a detailed
description of what the function or class does, including information about
parameters and return values.
**Example**:
```python
def greet(name):
"""
This function greets the person whose
name is passed as an argument.
Parameters:
name (str): The name of the person to
greet.
Returns:
None
"""
print(f"Hello, {name}!")
```
- In the example above, the docstring
describes what the `greet` function does, what parameters it expects, and what
it returns. Docstrings are useful for generating documentation and can be
accessed using the `help()` function in Python.
**Accessing Docstrings**:
```python
help(greet)
```
### 4.
**Commenting Out Code**
- You can use comments to temporarily
disable code without deleting it. This is useful for debugging or testing.
**Example**:
```python
x = 10
# y = 20
# This line is commented out and will not be executed
print(x)
```
- In the example above, the line `y = 20` is
commented out, so it will not be executed.
### Best
Practices for Using Comments
- **Keep
Comments Relevant**: Comments should accurately describe what the code does.
- **Avoid
Redundant Comments**: Don’t write comments that simply restate what the code
does.
- **Keep
Comments Concise**: Comments should be short and to the point.
- **Update
Comments**: Ensure comments are updated if the associated code changes.
- **Use
Docstrings for Functions**: Use docstrings for describing the purpose,
parameters, and return values of functions and classes.
###
Conclusion
Comments in
Python are a powerful tool for writing clear and maintainable code. They help
others (and your future self) understand the logic behind your code and ensure
that your codebase is easy to navigate and modify.