Forgot bit locker pin, forgot bit locker recovery key, 5 Easy ways to fix
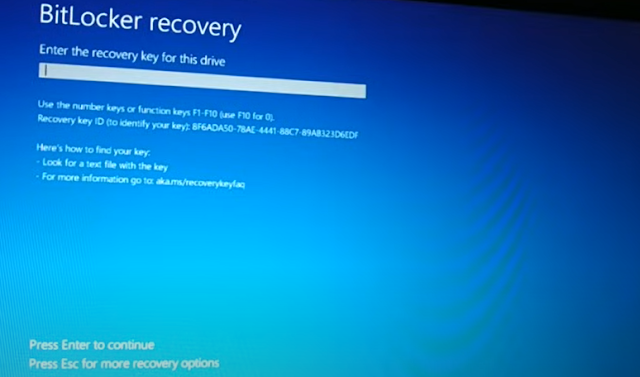
Scope in JavaScript refers to the accessibility of variables,
functions, and objects in different parts of your code. It determines where
these variables can be accessed or modified. Understanding scope is crucial for
writing clean, efficient, and bug-free code.
### Types of Scope
1. **Global Scope**
2. **Local/Function Scope**
3. **Block Scope**
### Global Scope
Variables declared outside of any function or block are in
the global scope. These variables are accessible from anywhere in the code.
```javascript
let globalVar = "I am a global variable";
function displayGlobalVar() {
console.log(globalVar); // Accessible here
}
displayGlobalVar(); // Outputs: I am a global variable
console.log(globalVar); // Accessible here as well
```
### Local/Function Scope
Variables declared within a function are in the local or
function scope. These variables are only accessible within that function and
cannot be accessed outside of it.
```javascript
function myFunction() {
let localVar =
"I am a local variable";
console.log(localVar); // Accessible here
}
myFunction();
console.log(localVar); // Error: localVar is not defined
```
### Block Scope
Variables declared with `let` or `const` within a block
(e.g., within `{}` braces, loops, or conditional statements) are block-scoped.
These variables are only accessible within that block.
```javascript
{
let blockVar =
"I am a block-scoped variable";
console.log(blockVar); // Accessible here
}
console.log(blockVar); // Error: blockVar is not defined
if (true) {
const conditionVar
= "I am also block-scoped";
console.log(conditionVar); // Accessible here
}
console.log(conditionVar); // Error: conditionVar is not
defined
```
### Var and Scope
The `var` keyword, unlike `let` and `const`, is not
block-scoped. Variables declared with `var` are either globally scoped or
function-scoped.
```javascript
if (true) {
var functionVar =
"I am function-scoped with var";
}
console.log(functionVar); // Accessible here
```
### Lexical Scope (Static Scope)
JavaScript uses lexical scoping, meaning that the scope of a
variable is determined by its position in the source code. Inner functions have
access to variables in their outer scope.
```javascript
function outerFunction() {
let outerVar =
"I am an outer variable";
function
innerFunction() {
console.log(outerVar); // Accessible here
}
innerFunction();
}
outerFunction(); // Outputs: I am an outer variable
```
### Closures
Closures are functions that remember their lexical scope even
when the function is executed outside that scope. This allows inner functions
to access variables from their outer function even after the outer function has
returned.
```javascript
function createCounter() {
let count = 0;
return function() {
count++;
return count;
};
}
let counter = createCounter();
console.log(counter()); // 1
console.log(counter()); // 2
console.log(counter()); // 3
```
### Shadowing
Shadowing occurs when a variable declared within a certain
scope (e.g., function or block) has the same name as a variable in an outer
scope. The inner variable shadows the outer one within its scope.
```javascript
let x = "global";
function shadowExample() {
let x =
"local";
console.log(x); //
"local"
}
shadowExample();
console.log(x); // "global"
```
### Hoisting
In JavaScript, variable and function declarations are hoisted
to the top of their containing scope during the compilation phase. However,
variables declared with `let` and `const` are hoisted but not initialized.
```javascript
console.log(hoistedVar); // undefined (hoisted but not
initialized)
var hoistedVar = "I am hoisted";
console.log(notHoistedVar); // ReferenceError: Cannot access
'notHoistedVar' before initialization
let notHoistedVar = "I am not hoisted";
```
### Example Usage
```javascript
let globalVar = "I am global";
function exampleFunction() {
let localVar =
"I am local";
if (true) {
let blockVar =
"I am block-scoped";
console.log(globalVar);
// Accessible
console.log(localVar); // Accessible
console.log(blockVar); // Accessible
}
console.log(globalVar); // Accessible
console.log(localVar); // Accessible
//
console.log(blockVar); // Error: blockVar is not defined
}
exampleFunction();
console.log(globalVar); // Accessible
// console.log(localVar); // Error: localVar is not defined
// console.log(blockVar); // Error: blockVar is not defined
```
### Summary
- **Global Scope**: Variables are accessible from anywhere in
the code.
- **Local/Function Scope**: Variables are accessible only
within the function they are declared.
- **Block Scope**: Variables declared with `let` and `const`
are accessible only within the block they are declared.
- **Lexical Scope**: Inner functions have access to variables
from their outer scope.
- **Closures**: Functions that retain access to their lexical
scope.
- **Shadowing**: Inner variables can shadow outer variables
with the same name.
- **Hoisting**: Variable and function declarations are moved to the top of their scope during compilation.